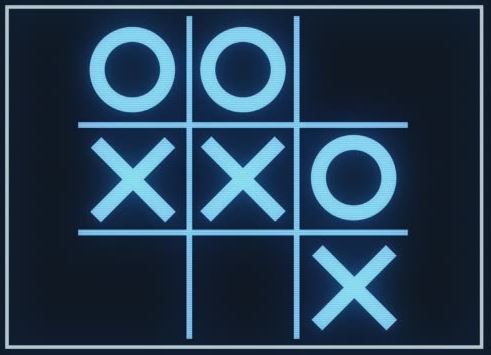
HTML
<div class="board" id="board">
<div class="cell" data-cell></div>
<div class="cell" data-cell></div>
<div class="cell" data-cell></div>
<div class="cell" data-cell></div>
<div class="cell" data-cell></div>
<div class="cell" data-cell></div>
<div class="cell" data-cell></div>
<div class="cell" data-cell></div>
<div class="cell" data-cell></div>
</div>
<div class="game-end" id="game-end">
<div class="warning-message" id="warning-message">
</div>
<div>
<button onclick="restart()">
Restart
</button>
</div>
</div>
CSS
body {
margin:0px;
padding:0px;
}
.board {
display: grid;
justify-content: center;
align-content: center;
grid-template-columns: repeat(3, auto);
}
.cell {
width: 40px;
height: 40px;
border: 1px solid #777777;
display: flex;
justify-content: center;
align-content: center;
position: relative;
}
.cell.x::before, .cell.x::after {
content: '';
width: 5px;
height: 35px;
position: absolute;
background: #000000;
}
.cell.x::before {
transform: rotate(45deg);
}
.cell.x::after {
transform: rotate(-45deg);
}
.cell.circle::before, .cell.circle::after {
content: '';
position: absolute;
border-radius: 50%;
}
.cell.circle::before {
width: 35px;
height: 35px;
background: #000000;
}
.cell.circle::after {
width: 25px;
height: 25px;
background: #ffffff;
}
.game-end {
display: none;
position: fixed;
top:0px;
left: 0px;
bottom:0px;
right: 0px;
z-index:101;
background-color: rgba(0,0,0,0.9);
justify-content: center;
align-content: center;
color: white;
font-size: 2rem;
}
.game-end.show {
display: flex;
}
JavaScript
const cellElem = document.querySelectorAll('.cell');
let circleTurn;
const winCons = [
[0,1,2],
[3,4,5],
[6,7,8],
[0,3,6],
[1,4,7],
[2,5,8],
[0,4,8],
[2,4,6]
]
startGame();
function startGame() {
circleTurn = false;
cellElem.forEach(cell => {
cell.addEventListener('click', handleClick, {once: true})
})
}
function handleClick(e) {
//console.log ('Cell Clicked.');
//placemark
//check for win
//check for draw
//switch turns
const cell = e.target;
console.log(e.target);
const currentClass = circleTurn ? 'circle' : 'x';
placeMark(cell, currentClass);
if(checkwin(currentClass)) {
console.log(currentClass + 'Winner');
endGame(false);
}
else if (isDraw()){
endGame(true);
}
else{
swapTurns();
}
}
function placeMark (cell, currentClass) {
cell.classList.add(currentClass);
}
function swapTurns() {
circleTurn = !circleTurn;
}
function checkwin(currentClass){
return winCons.some(combi => {
return combi.every(index => {
return cellElem[index].classList.contains(currentClass)
})
})
}
function endGame(draw) {
if(draw){
document.getElementById('warning-message').innerHTML = 'Draw!';
}
else {
document.getElementById('warning-message').innerHTML = `${circleTurn ? "0 Wins" : "X Wins"}`;
}
document.getElementById('game-end').classList.add('show');
}
function isDraw() {
return [...cellElem].every(cell => {
return cell.classList.contains('x') || cell.classList.contains('circle');
})
}
function restart() {
cellElem.forEach(cell => {
cell.classList.remove('x');
cell.classList.remove('circle');
cell.removeEventListener('click', handleClick);
});
document.getElementById('warning-message').innerHTML = '';
document.getElementById('game-end').classList.remove('show');
startGame();
}
Working Example – https://jsfiddle.net/deepak104080/L7e15dbu/101/